9.Classes and Objects
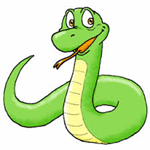
9.1 Classes and objects: Programmer-defined types, Attributes, Rectangles, Instances as return
values, Objects are mutable, Copying,
9.2 Classes and functions: Time, Pure functions, Modifiers, Prototyping versus planning,
9.3 Classes and methods: Object-oriented features, Printing objects, Another example, A more
complicated example,The __init__ method, The str method, Operator overloading, Type-based
dispatch, Polymorphism, Interface and implementation,
9.1 Classes and objects
Python is an object oriented programming language. Class is a abstract data type which can be defined as a template or blueprint that describes the behavior / state that the object of its type support.
An object is an instance of class . Objects have states and behaviors.
Example: A dog has states – color, name, breed as well as behaviors – wagging the tail, barking, eating.
Object is simply a collection of data (variables) and methods (functions) that act on those data. The process of creating this object is called instantiation
Defining a Class in Python :
We define a class using the keyword class. The first string is called docstring and has a brief description about the class. Although not mandatory, this is recommended. A class attributes are data members( variables) and functions.
Syntax :
The class statement creates a new class definition. The name of the class immediately follows the keyword class followed by a colon as follows −
class ClassName:
“””Optional class documentation string’ class_suite”””
class_suite
•The class has a documentation string, which can be accessed via ClassName.__doc__.
•The class_suite consists of all the component statements defining class members, data attributes and method.
Example 1
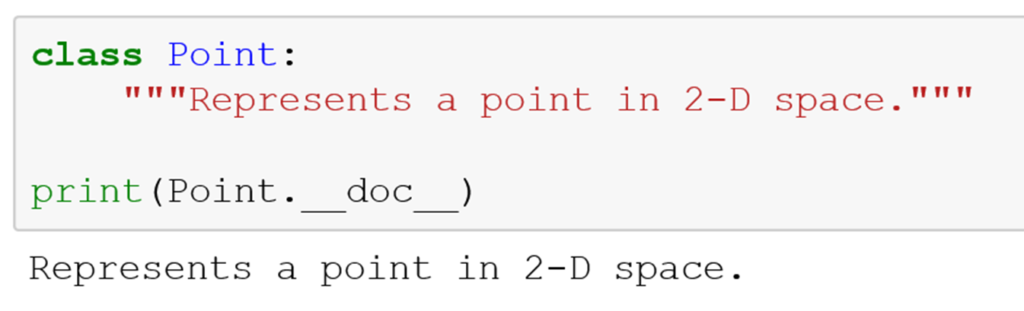
The header indicates that the new class is called Point.
The body is a docstring that explains what the class is for. You can define variables and methods inside a class definition.
Creating an Object in Python:
•Class can be used to create new object instances (instantiation) of that class.
•The procedure to create an object is similar to a function call.
Example :
blank = Point()
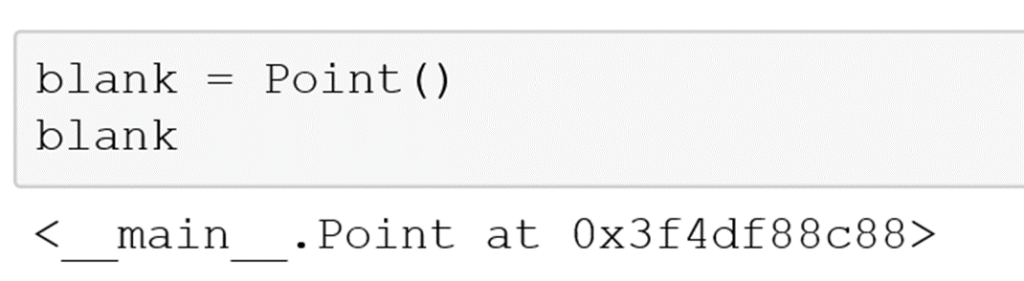
The return value is a reference to a Point object, which we assign to blank.
Example1 : Creating an object of Point Class
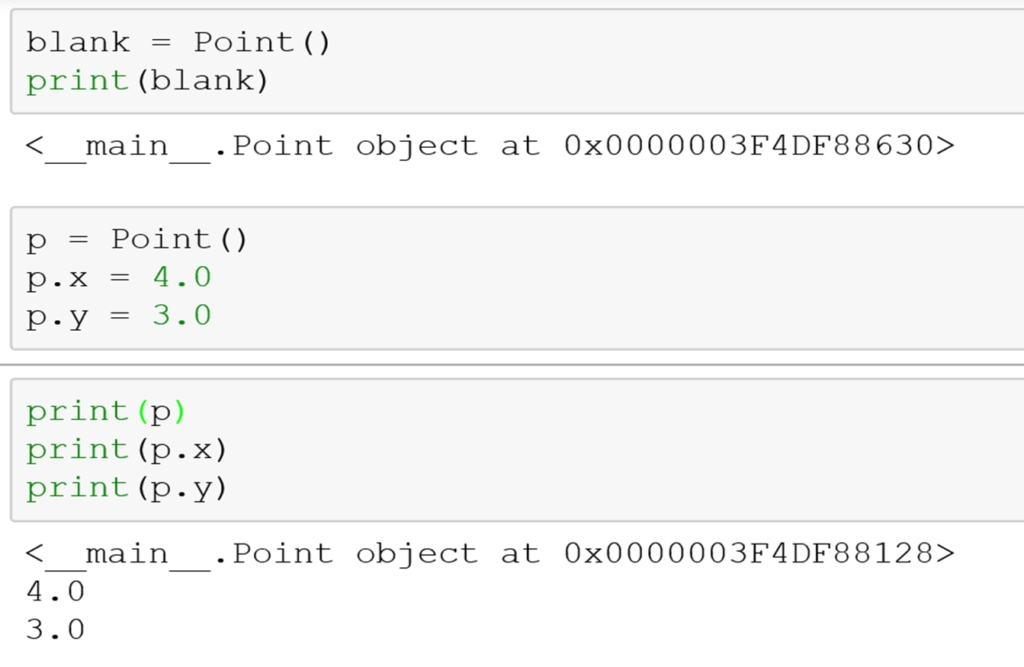
Example 2 : Creating Employee class and its objects obj1 and obj2
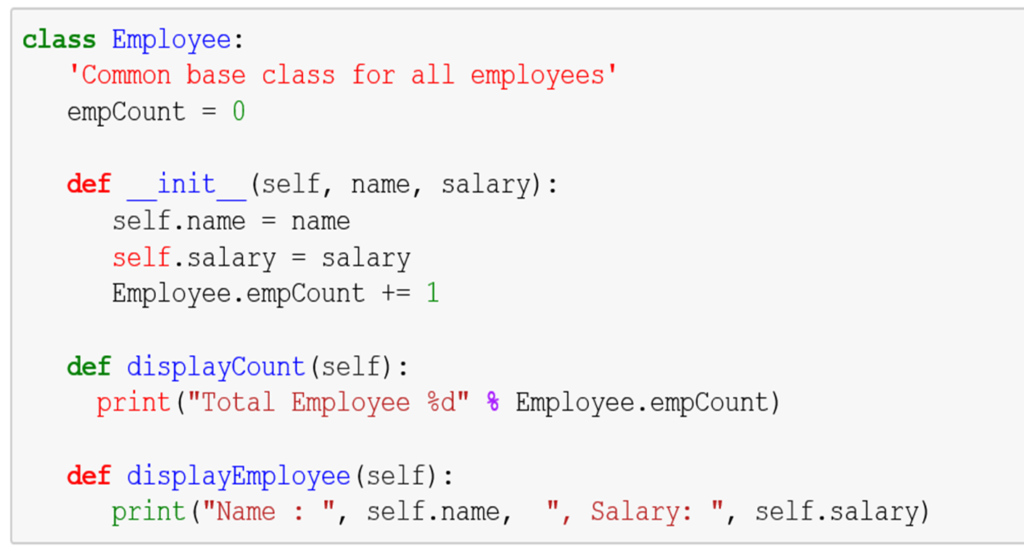
•The variable empCount is a class variable whose value is shared among all instances of a this class. This can be accessed as Employee.empCount from inside the class or outside the class.
•The first method __init__() is a special method, which is called class constructor or initialization method that Python calls when you create a new instance of this class.
•We can declare other class methods like normal functions with the exception that the first argument to each method is self.
•Python adds the self argument to the list for you; you do not need to include it when you call the methods.
Creating Instance Objects :
•To create instances of a class, you call the class using class name and pass in whatever arguments its __init__method accepts
emp1 = Employee(“Raju”, 2000)
emp2 = Employee(“Swamy”, 5000)
Accessing Attributes
We can access the object’s attributes using the dot operator with object. Class variable would be accessed using class name as follows −
emp1.displayEmployee()
emp2.displayEmployee()
print (“Total Employee %d” % Employee.empCount)
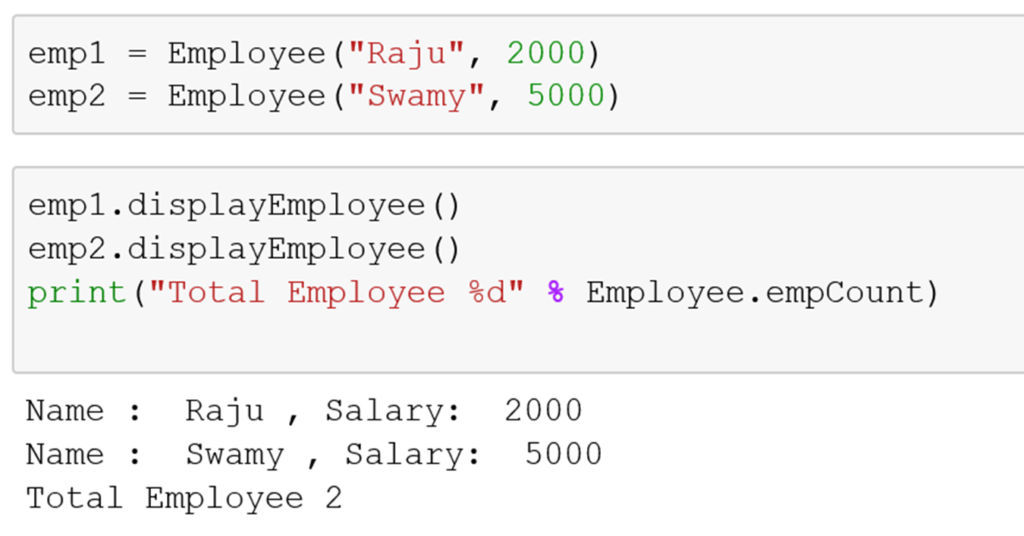
Entire program put together
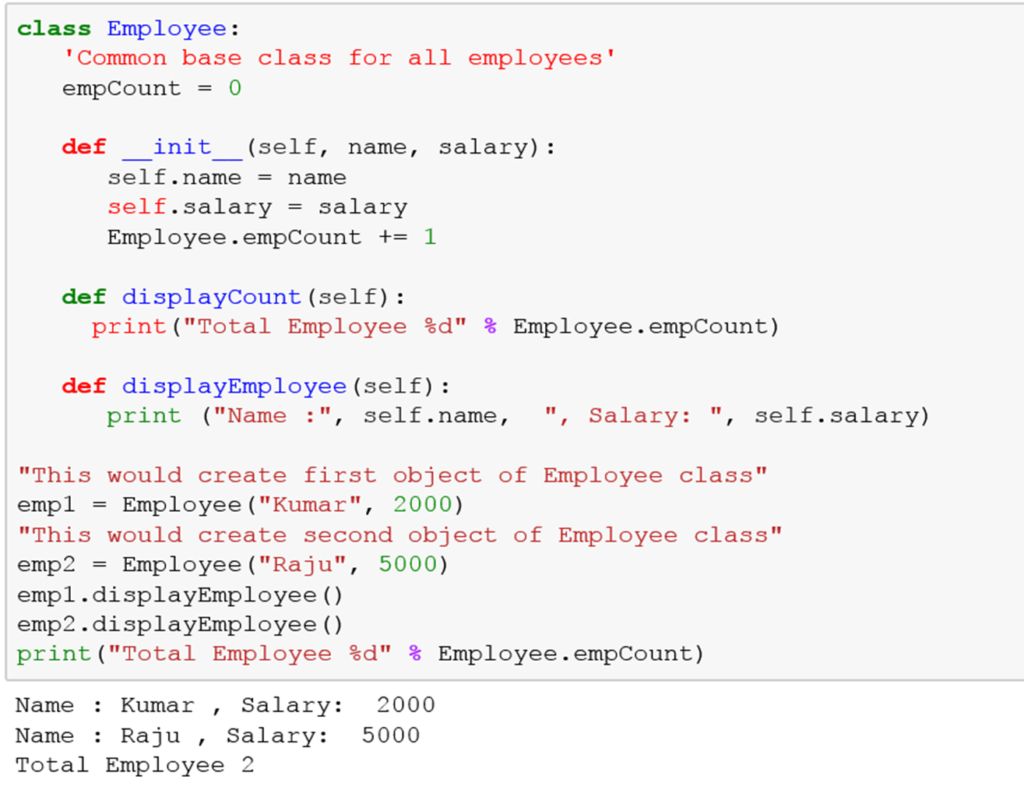
Example 3 : Rectangle
Rectangle can be created using following two possibilities:
–You could specify one corner of the rectangle (or the center), the width, and the height.
–You could specify two opposing corners.
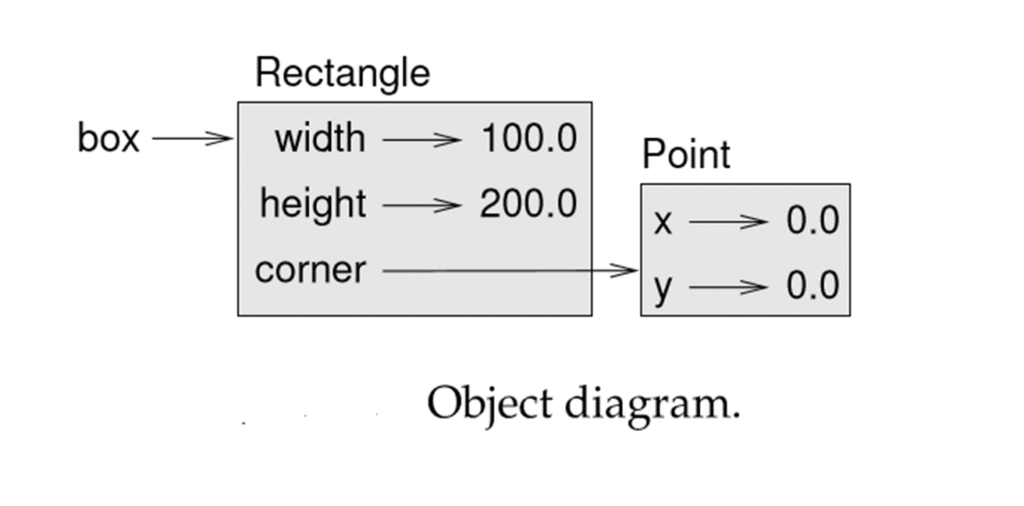
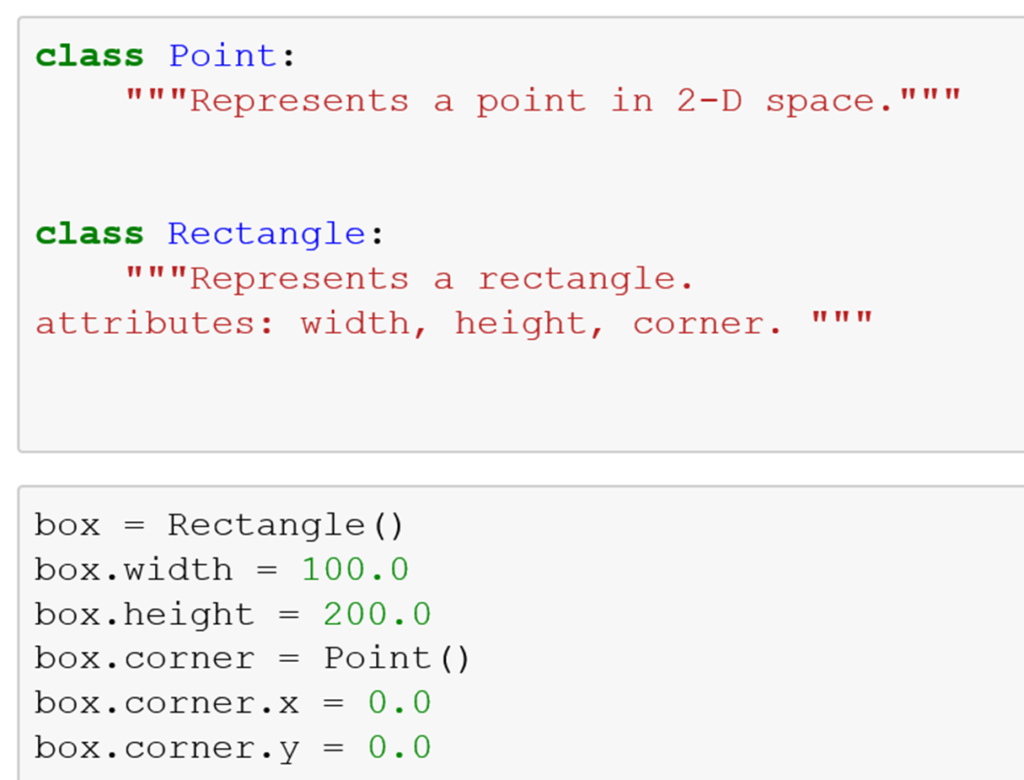
Instances as return values
Functions can return instances. For example, find_center takes a Rectangle as an argument and returns a Point that contains the coordinates of the center of the Rectangle:
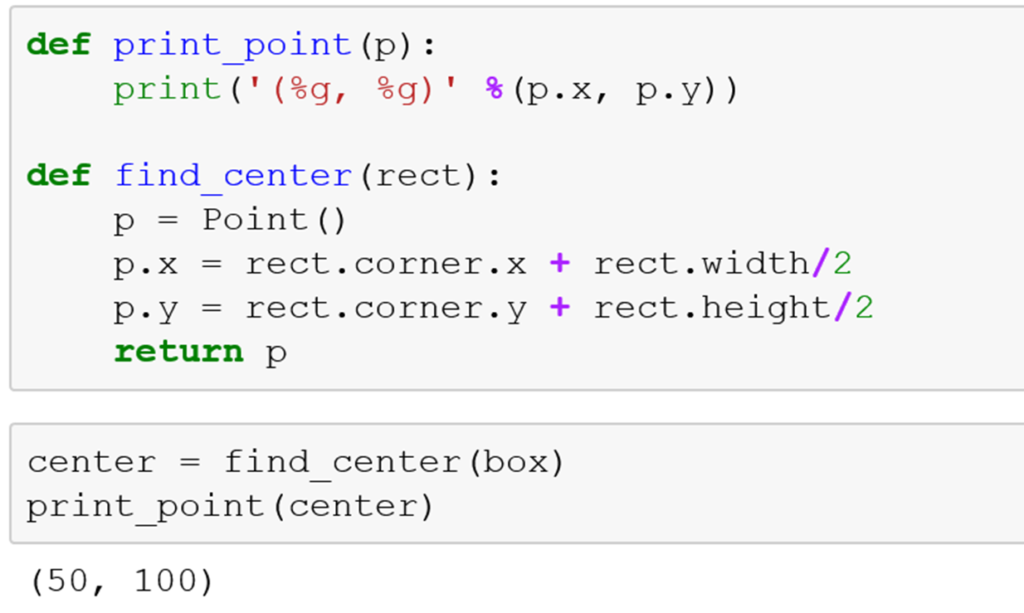
Objects are mutable
You can change the state of an object by making an assignment to one of its attributes. For example, to change the size of a rectangle without changing its position, you can modify the values of width and height:
•box.width = box.width + 50
•box.height = box.height + 100
Modifying through functions
You can also write functions that modify objects.For example, grow_rectangle takes a Rectangle object and two numbers, dwidth and dheight, and adds the numbers to the width and height of the rectangle:
def grow_rectangle(rect, dwidth, dheight):
rect.width += dwidth
rect.height += dheight
Copying
Copying an object is often an alternative to aliasing. The copy module contains a function called copy that can duplicate any object as illustrated below :
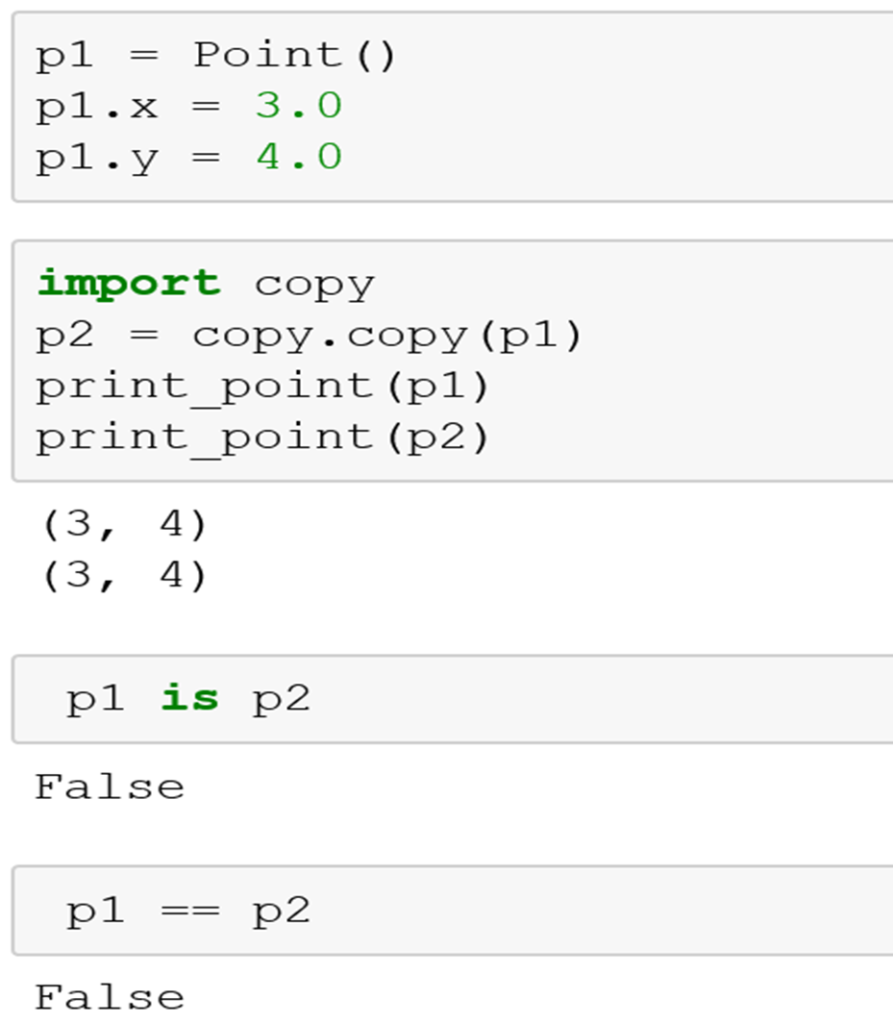
9.2 Classes and Functions
•Here functions means User Defined Functions and they are defined outside the class. Consider a definition of Class
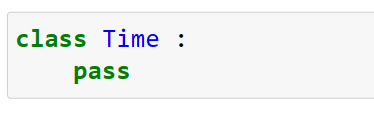
New Objects of Class the class can be created as illustrated below :
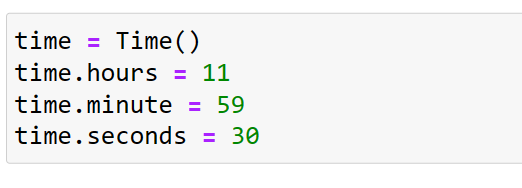
•User defined Function to display the attribute of object say ob.
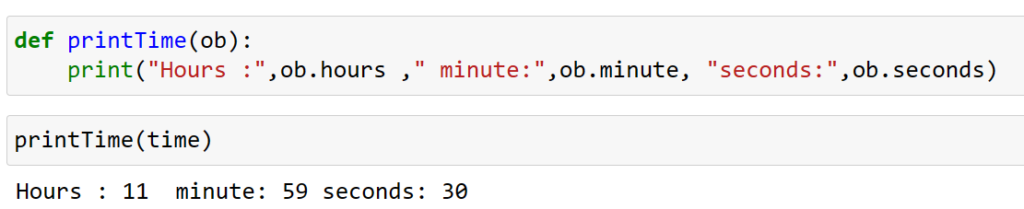
In the above example user defined function printTime(ob) is defined outside the class and it is called to print the value object attributes as illustrated above.
There are two types of functions used for classes :
- Pure functions
- Modifiers
1. Pure Functions
The function which creates new object , initiates its attributes and returns a reference to the new object is called a pure function. It is called pure function because it does not modify any of the objects passed to it as arguments and it has no side effects, such as displaying value or getting user input.
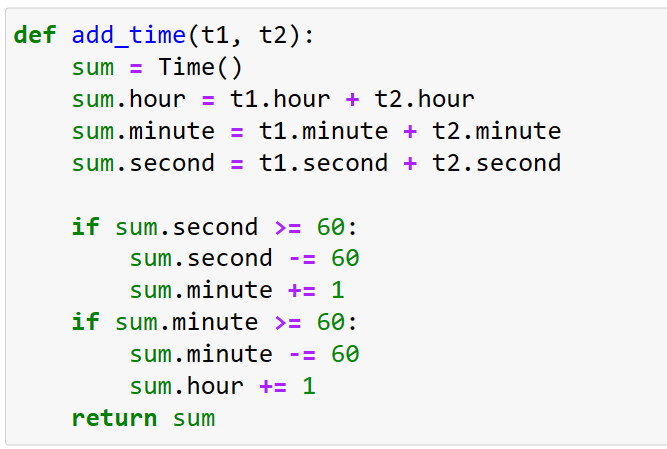
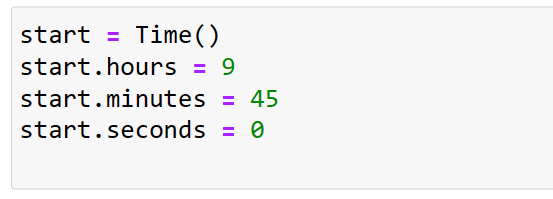
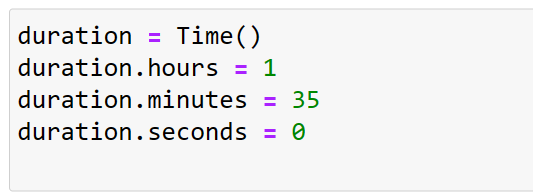
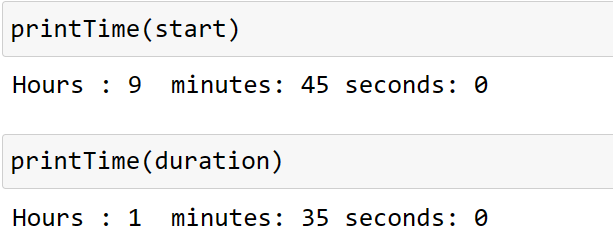
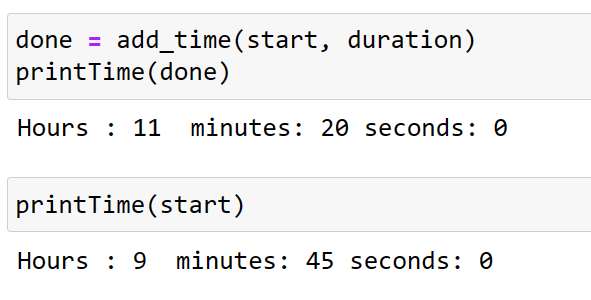
2. Modifiers
The functions which are used to modify one or more of the objects its gets as arguments are called modifiers. Most modifiers are fruitful.
Example:
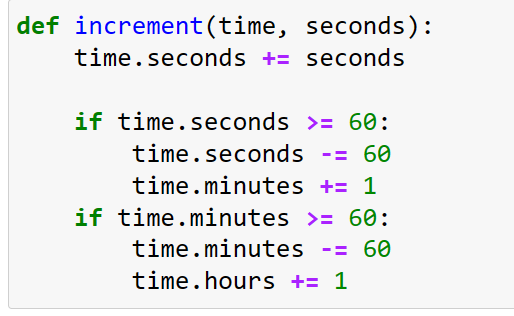
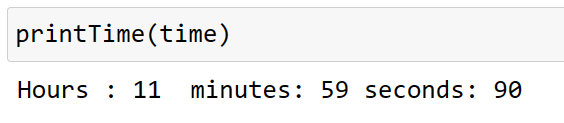
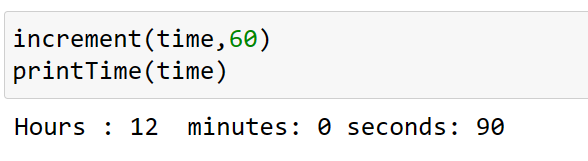
Prototype and Planned Development
Prototype Development
•Prototype development involves
–Writing a Rough draft of programs ( or prototype)
–Performing a basic calculation
–Testing on a few cases , correcting flaws as we found them.
•Prototype development leads code complicated
Planned Development
High level insight into the problem can make the programming much easier
Example : A time object is really a three digit number in base 60. The second component is the “Ones Column” the minute component is the “sixties column” and the hour component is the thirty six hundred column.
Examples of Planned Development :
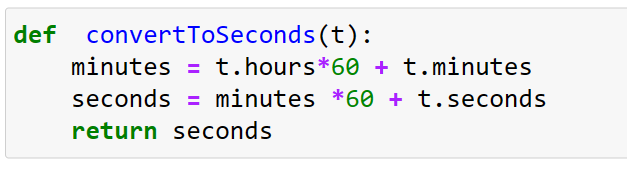
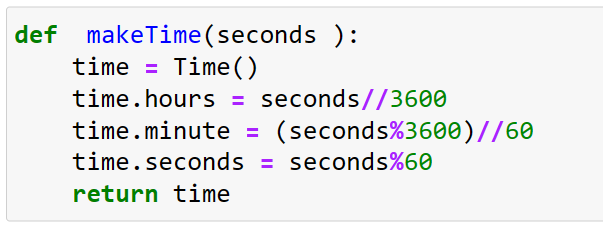

Classes and Methods
Object Oriented Features : Python is an object-oriented programming language, which means that it provides features that support object-oriented programming, which has these defining characteristics:
–Programs include class and method definitions.
–Most of the computation is expressed in terms of operations on objects.
–Objects often represent things in the real world, and methods often correspond to the ways things in the real world interact
Printing Objects

To call this function, you have to pass a Time object as an argument:
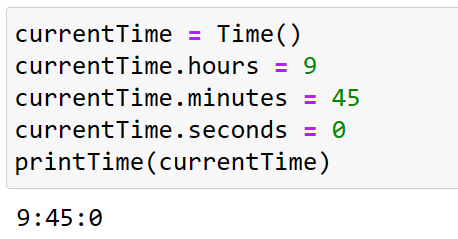
Methods versus Functions
Methods are just like functions with two differences :
–Methods are defined inside a class definition in order to make the relationship between the class and the method explicit
–The syntax for invoking a method is different from the syntax for calling a function.
Transforming functions into methods
•The function definition must be done within the class definition
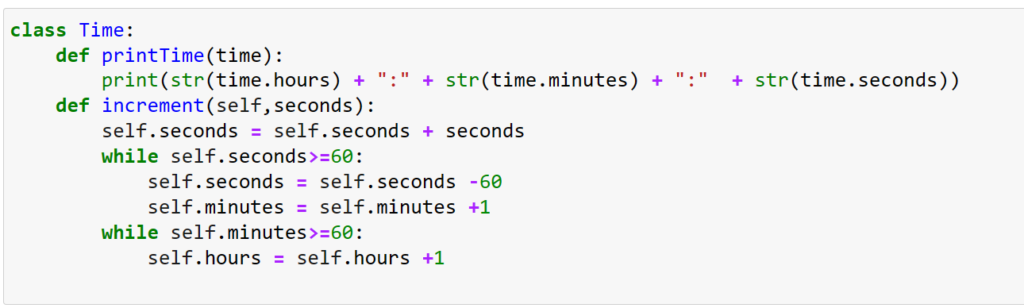
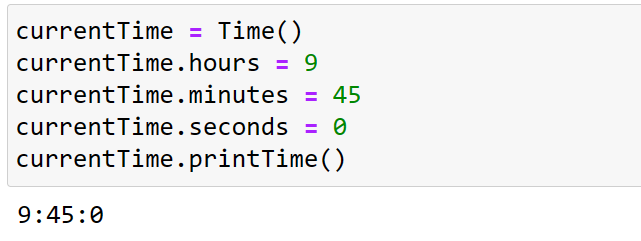
Invoking increment as a method
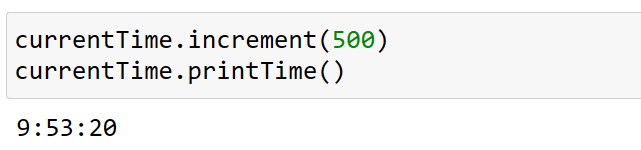
Optional Arguments
•In python we can write user defined functions with optional argument lists.
Example :
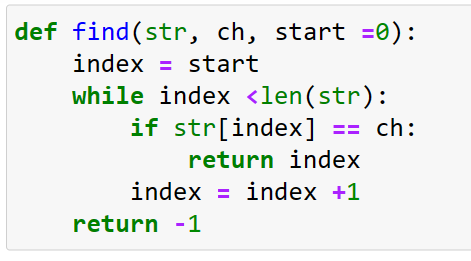
•The third parameter start is optional because a default value 0 is provided.
•If we invoke find with only two argument it uses the default value and start from the beginning of the string:
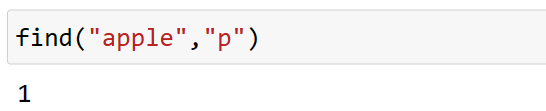
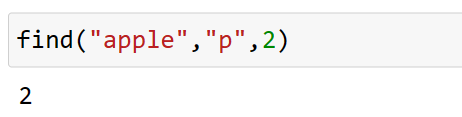
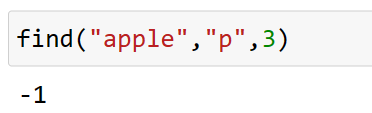
Initialization __init__ method
•The initialization method is a special method that is invoked when an object is created.The name of this method is : __init__ (two underscore characters followed by init and then two more underscore)
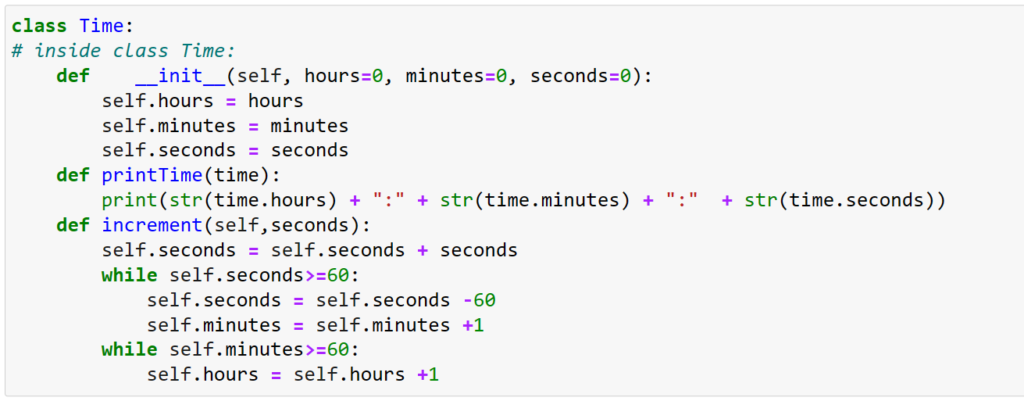
Invoking methods
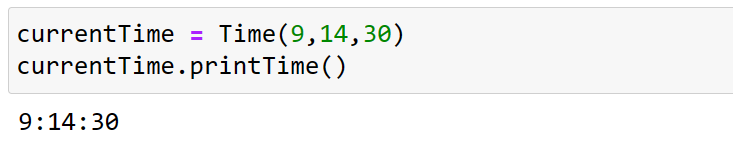
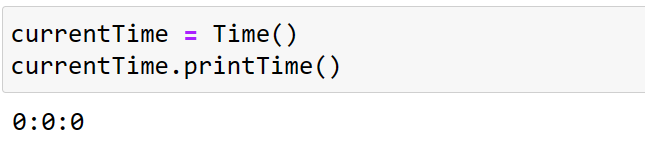
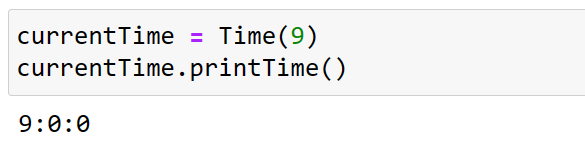
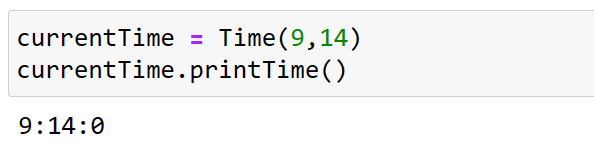
__str__ method
This method returns the string representation of the object. This method is called when print() or str() function is invoked on an object.
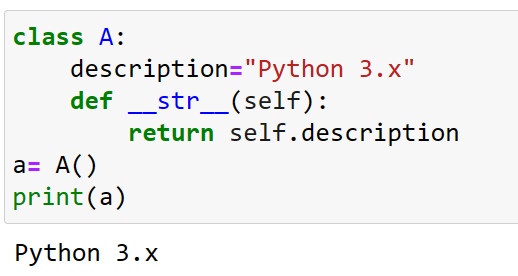
Example :
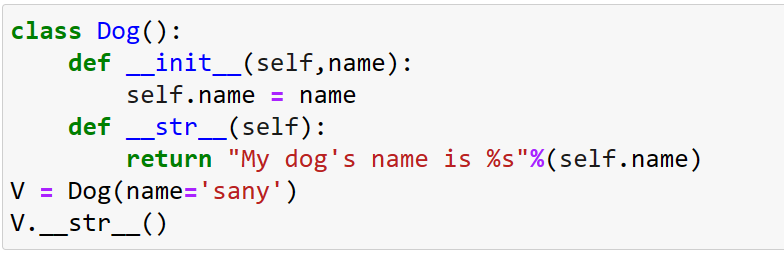

Method Overriding
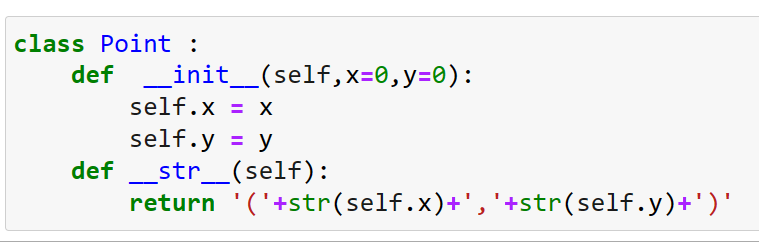
•If a class provides a method __str__ it overrides the default behavior of the Python built in str function
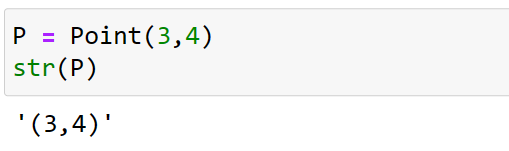
Operator Overloading
Operator overloading is one of the feature of OOP language which make possible to change the definition of the build in operators when they are applied to user defined type. It is especially useful when defining new mathematical types.
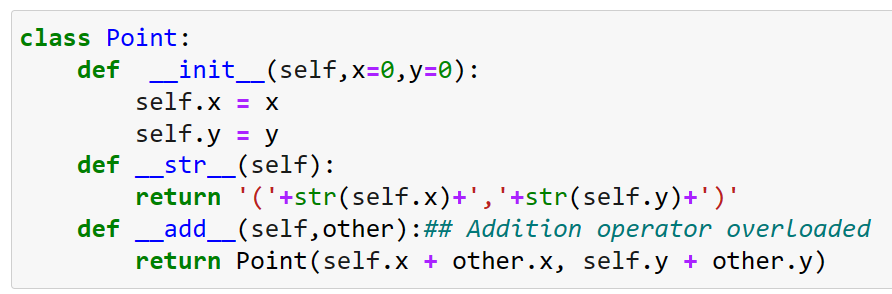
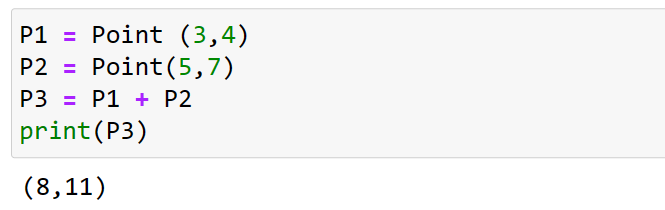
Type based dispatch
•Type dispatch allows you to change the way a function behaves based upon the input types it receives.
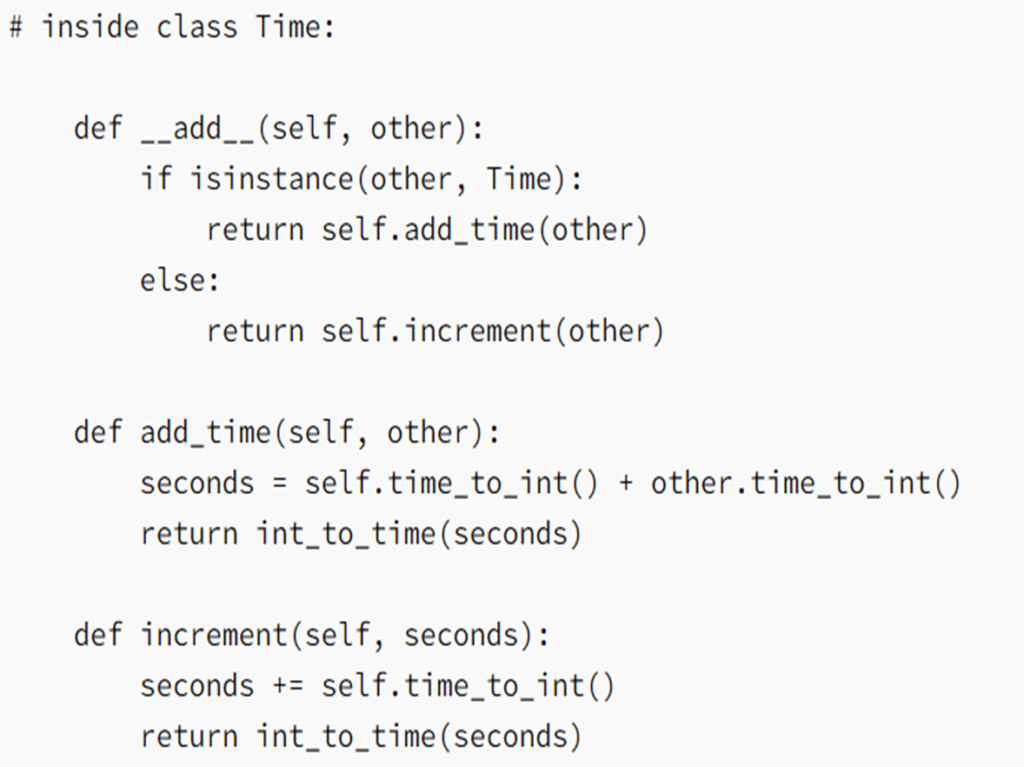
If other is a Time object, __add__ invokes add_time. Otherwise it assumes that the parameter is a number and invokes increment. This operation is called a type-based dispatch because it dispatches the computation to different methods based on the type of the arguments. Here are examples that use the + operator with different types:
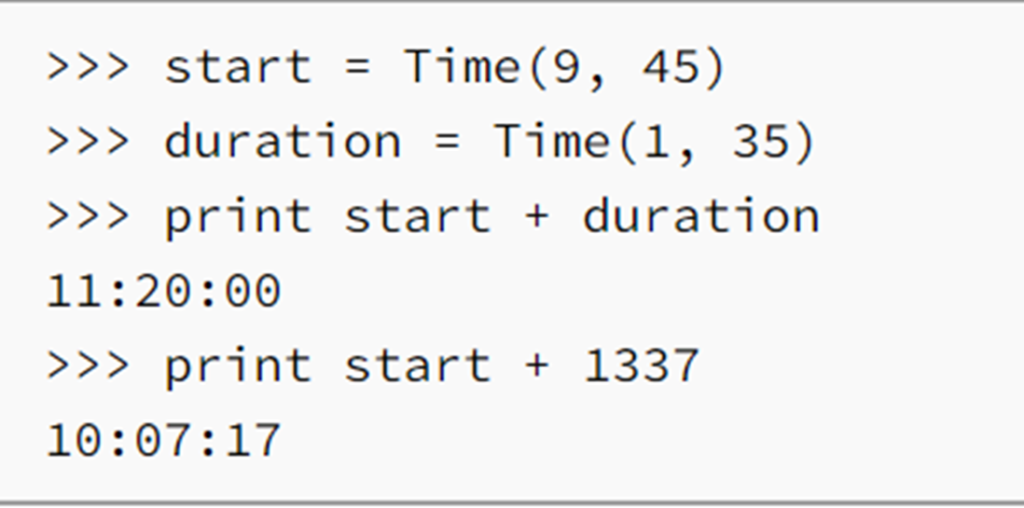
Unfortunately, this implementation of addition is not commutative. If the integer is the first operand, you get

The problem is, instead of asking the Time object to add an integer, Python is asking an integer to add a Time object, and it doesn’t know how to do that. But there is a clever solution for this problem:
the special method __radd__, which stands for “right-side add.” This method is invoked when a Time object appears on the right side of the + operator.
Here’s the definition:
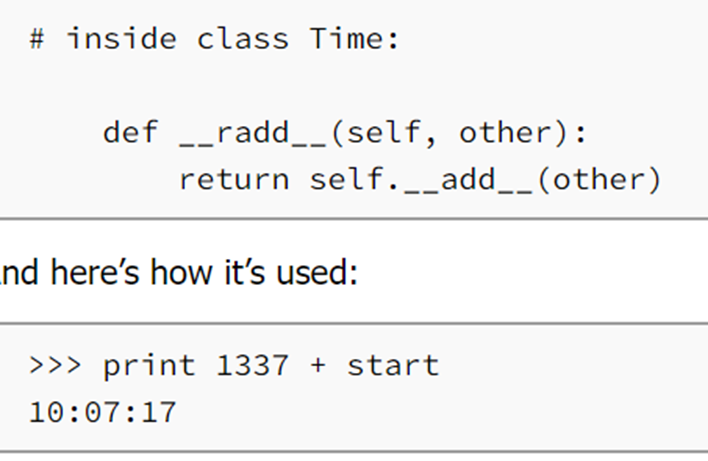
Polymorphism
The literal meaning of polymorphism is the condition of occurrence in different forms. Polymorphism refers to the use of a single type entity (method, operator or object) to represent different types in different scenarios.
A polymorphic function can operate on more than one type . If all the operations in a function can be applied to a type , then the entire function can also be applied to a type.
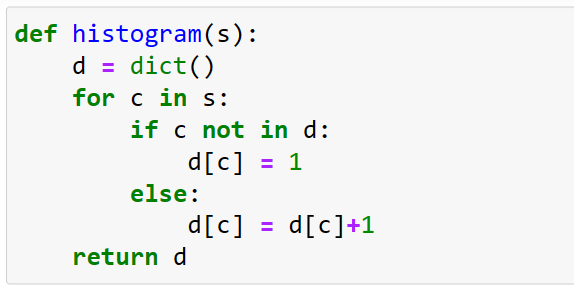
This function also works for lists, tuples, and even dictionaries, as long as the elements of
s are hashable, so they can be used as keys in d.
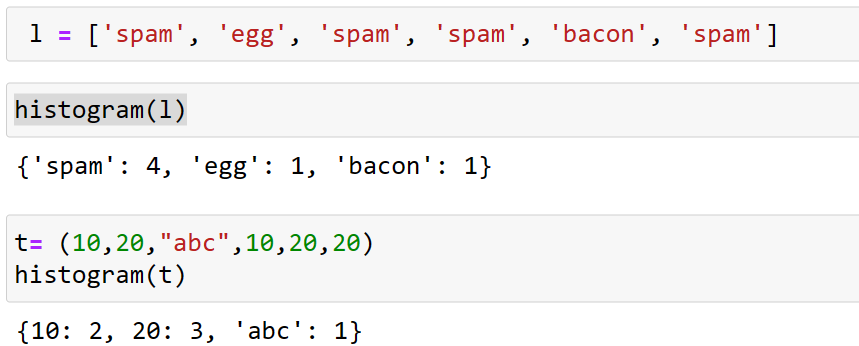
Functions that work with several types are called polymorphic. Polymorphism can facilitate code reuse. For example, the built-in function sum, which adds the elements of a sequence, works as long as the elements of the sequence support addition
Practice Questions
- What is class ? How do we define a class in python? How to instantiate the class and how class members are accessed?
- Write a python program that uses a datetime module within a class , takes a birthday as input and prints users’ age and the number of days , hours , minutes and seconds until their next birthday .
- Explain __init__ and __str__ methods .
- Explain operator overloading with examples .
- What are polymorphic functions ? Explain with code snippet
- Illustrate the concept of inheritance with example.
- Define classes and objects in Python. Create a class called Employee and initialize it with
employee id and name . Design methods to :
a. set_Age_ to assign salary to the employee
b. Display to display all information of the employee. - Illustrate the concept of modifier with Python Code
- Explain init and str method with an example Python Program .
- Define polymorphism ? Demonstrate polymorphism with function to find histogram to count the number of times each letter appears in a word and in a sentence.
- Illustrate the concept of pure function with Python Code.
- Define Class Diagram . Discuss the need for representing class relationships using class diagram with suitable example.
- Explain the init and str method with an example python program
- Write a python program that uses datetime module within a class , takes a birthday as input and prints the age and number of days , hours , minutes and second .
- What is a pure function ? Illustrate with an example python program
- Define Polymorphism. Demonstrate polymorphism with function to find histogram to count the numbers of times each letters appears in a word and in sentence.
- What is type based dispatch ? Illustrate with python program
- Create a class called
Person
with attributesname
,age
, andgender
. Add a methodgreet()
that prints “Hello, my name is [name]” when called. - Create a class called
Rectangle
with attributeswidth
andheight
. Add methodsarea()
andperimeter()
that return the area and perimeter of the rectangle, respectively. - Create a class called
BankAccount
with attributesaccount_number
andbalance
. Add methodsdeposit()
andwithdraw()
that modify the balance of the account. - Create a class called
Animal
with attributesname
andspecies
. Add a methodspeak()
that prints “Hello, my name is [name]” when called. - Create a class called
Student
with attributesname
,age
, andmajor
. Add methodsstudy()
andsleep()
that print “I am studying” and “I am sleeping”, respectively. - Create a class called
Car
with attributesmake
,model
, andyear
. Add methodsaccelerate()
andbrake()
that modify the speed of the car. - Create a class called
Person
with attributesname
,age
, andaddress
. Add a methodchange_address()
that modifies the address of the person. - Create a class called
Circle
with attributeradius
. Add methodsarea()
andcircumference()
that return the area and circumference of the circle, respectively. - Create a class called
Book
with attributestitle
,author
, andpages
. Add a methodread()
that prints “I am reading [title]” when called. - Create a class called
Rectangle
with attributeswidth
andheight
. Add a methoddraw()
that draws a rectangle with the given width and height on the screen. - Create a class called
Student
with attributesname
,roll_number
,marks_list
, andnumber_of_subjects
. Add the following methods to the class:__init__()
method to initialize the attributes of the class.get_details()
method to print the name and roll number of the student.get_average_marks()
method to calculate and return the average marks of the student.- Create an object and display details.