4.Lists
Lists: The List Data Type, Working with Lists, Augmented Assignment Operators, Methods, Example Program: Magic 8 Ball with a List, List-like Types: Strings and Tuples, References,
The most basic data structure in Python is the sequence. Each element of a sequence is assigned a number – its position or index. The first index is zero, the second index is one, and so forth. Like a string, a list is a sequence of values. In a string, the values are characters; in a list, they can be any type. The values in list are called elements or sometimes items. There are several ways to create a new list; the simplest is to enclose the elements in square brackets ([ and ]):
List Data type:
A list is a value that contains multiple values in an ordered sequence. The term list value refers to the list itself (which is a value that can be stored in a variable or passed to a function like any other value), not the values inside the list value. A list value looks like this: [‘cat’, ‘bat’, ‘rat’, ‘elephant’].
List is a sequence of values of any type. The values are called elements/items enclosed within square brackets.
Example : l1= [1,2,3] and l2 =[“ab”,19,20.75,”xyz”]
4.1 Working With List
What are the different ways to create a list .
- Using pair of square brackets [ ]
- Using built in function like list(), append() and extend()
4.1.1: Creation of List using pair of square brackets [ ]
List can be created using pair of square brackets [] , by providing the name of the list and initializing it with values. List may be empty or with the set of values. Following examples illustrates the creation of varieties of lists:
Creation of Empty list :

Creation of List with values

4.1.2.Using built in functions
- We can add one item to a list using list() , append() method or add several items using extend() method.

4.1.2 Lists are Mutable
Unlike strings , lists are mutable because you can change the order of items in a list or reassign an item in a list using index as illustrated below:

4.1.3 List Concatenation
+ : operator concatenates lists

4.1.4 List Replication
*: operator repeats a list a given number of times

4.1.5 Removing values from lists with del statement

4.1.6 Accessing List values (Getting individual values in lists with indexes.)
One can think List as a relationship between indices and elements. This relationship is called a mapping, each index ‘maps to’, one of the elements. Python Supports both positive and negative indexing of list elements as illustrated below.


List indices work the same way as string indices. Any integer expression can be used as an index. If you try to read or write an element that does not exist, you get an Index Error. If an index has a negative value, it counts backward from the end of the list.
Example :
Accessing through Positive indexes



Accessing through Negative indexes


Index Error

4.1.7 Traversing a list using for Loop
The most common way to traverse the elements of a list is with a for loop. The syntax and example is as illustrated in the example below

This loop traverses the list and updates each element. len returns the number of elements in the list. range returns a list of indices from 0 to n − 1, where n is the length of the list.
Note 1 : A for loop over an empty list never executes the body.

Note 2 : Although a list can contain another list, the nested list still counts as a single element. The length of this list is four:

4.1.8 List Slicing /Getting Sublists with Slices
List slicing is a process of accessing a part/subset / range of elements in a list. Slicing is done using colon (:) operator .
Syntax for slicing :
list_name [ start: stop: steps]
The start parameter is a mandatory parameter, whereas the stop and steps are both optional parameters. The start represents the index from where the list slicing is supposed to begin.
Its default value is 0, i.e. it begins from index 0.The stop represents the last index up to which the list slicing will go on.
Its default value is (length(list)-1) or the index of last element in the list.The steps represents the number of steps,i.e after every n number of steps,
the start index is updated and list slicing is performed on that index.
Examples :
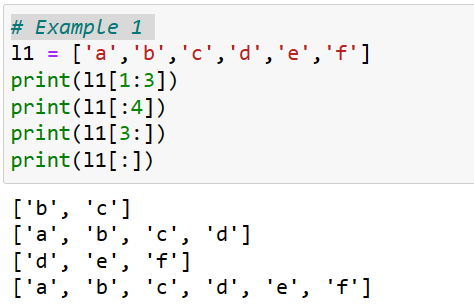

Using for Loops with lists
For loop can be used to visit all / specified elements of list as illustrated below:
i) Visiting all elements of List using For loop


Usage of in and not in membership operators in list


Multiple Assignment


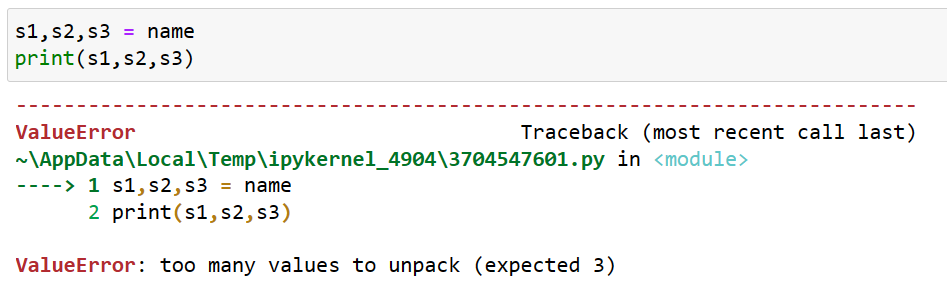
Augmented Assignment Operator (Op =):
Consider the following expression

As a shortcut one can use the augumented assignment operator + = to do the same thing as above expressions as illustrated below :

There are augmented assignment operators for the +,-,* ,/ and % operators described in table below :
Augmented assignment operator | Equivalent assignment statement |
x+=1 | x=x+1 |
x-=1 | x=x-1 |
x*=1 | x=x*1 |
x/=1 | x=x/1 |
x%=1 | x=x%1 |
The += operator can also do string and list concatenation and the *= operator can do string and list replication as illustrated below :


List Methods
The list data type has several useful methods for finding , adding removing and otherwise manipulating values in a list. Some of the list methods are :
- index()
- append()
- insert()
- remove()
- del()
- pop()
- sort()
1. index () method : Index method returns the position / index number of element in a list.

2.append() method : To add new values to a list , one cane use the append() and insert() methods as illustrated below :

3. Insert() method : insert() method inserts a given element at a given position in a list.

4. remove () method : The remove() method is used to delete the element when its value is known.The remove() method removes the first occurrence of the element with the specified value.

5. del() method : The del statement is good to use when you know the index of the value you want to remove from the list.


6. pop() method : If you know the index of the element you want , you can use pop().

7. sort() method : Lists of number values or list of strings can be sorted with the sort() method as illustrated below :
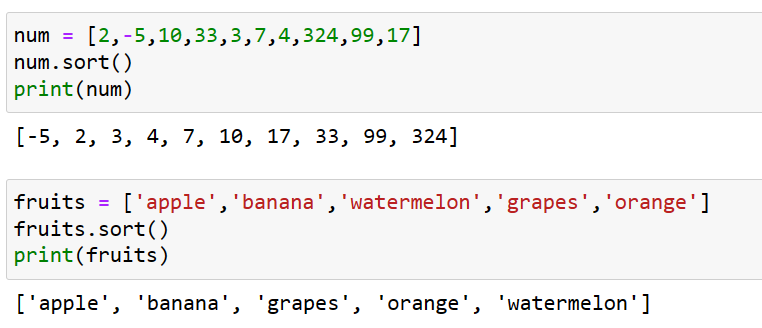
By default sort() method sorts in ascending order. It can be used to sort the elements in reverse order as illustrated below :

Lists and Functions
There are a number of built in functions that can be used on lists that allow you can be used on lists that allow you to quickly look through a list without writing your own loops. Some of the functions used with lists are len(), max(), min(),sum(),etc. The sum() function only works when the list elements are number . The other functions max(), len(),etc., work with lists of strings and other types that can be comparable.



Questions
- What is list ? Explain the concepts of list creation and list slicing with examples.
- Define Tuple, List, Strings and Dictionary in Python.
- Explain why lists are called Mutable.
- Discuss the following List operations and functions with examples :
- Accessing ,Traversing and Slicing the List Elements
- + (concatenation) and * (Repetition)
- append, extend, sort, remove and delete
- split and join
- Explain the methods of list data type in Python for the following operations with suitable code snippets for each.
- a. Adding values to a list
- b. Removing values from a list
- c. Finding a value in a list
- d. Sorting the values in a list
- What is list ? Explain the concept of slicing and indexing with proper examples.
- For a given list num = [45,22,14,65,97,72] write a python program to replace all the integers divisible by 3 with”ppp” and all integers divisible by 5 with “qqq” and replace all the integers divisible by both 3 and 5 with” pppqqq” and display the output.
- What are the different LIST methods supported in python . Illustrate all the methods with an example.